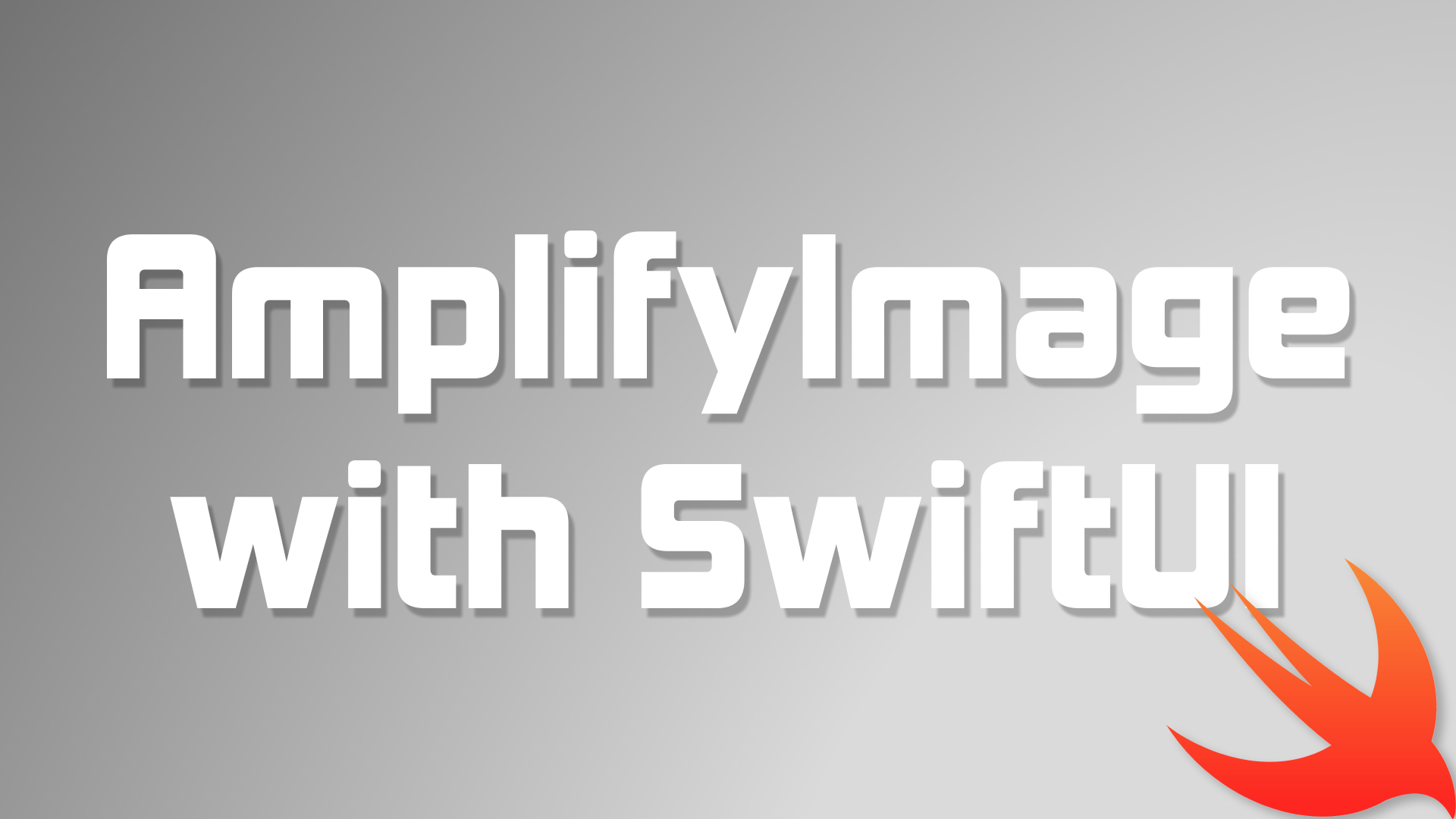
How to Display Images from AWS Amplify Storage on iOS Using AmplifyImage and SwiftUI
If you are an iOS developer looking to display images from AWS Amplify Storage in your application, AmplifyImage is the perfect library to use. AmplifyImage is a Swift package that simplifies the process of fetching and displaying images from AWS Amplify Storage in your iOS app. In this blog post, we will walk you through the steps to use AmplifyImage and AWS Amplify Storage with SwiftUI.
Step 1: Installing AmplifyImage
Once you have set up AWS Amplify Storage in your iOS project, you can install AmplifyImage by adding it to your project's dependencies. To do this, follow these steps:
- Open your project in Xcode.
- Click on File -> Swift Packages -> Add Package Dependency.
- In the search bar, enter "amplify-image" and select the package from the list.
- Click on "Add Package" to add AmplifyImage to your project.
Step 2: Displaying Images from AWS Amplify Storage with AmplifyImage and SwiftUI
With AmplifyImage installed, you can now display images from AWS Amplify Storage in your SwiftUI views. Follow these steps to display an image using AmplifyImage:
- Import the AmplifyImage package at the top of your SwiftUI view:
import AmplifyImage
- Use the AmplifyImage view to display the image:
AmplifyImage(key: "path/to/image.jpg")
In the above code, replace "path/to/image.jpg" with the path to your image in AWS Amplify Storage.
Step 3: Adding Placeholder and Error Images
You can use placeholders and error images to provide a better user experience when the image is not available or when it is still loading. Follow these steps to add a placeholder and error image:
Use the AmplifyImage view to display the image:
AmplifyImage(key: "path/to/image.jpg", placeholder: Image(systemName: "photo"), error: Image(systemName: "exclamationmark.triangle"))
In the above code, Image(systemName: "photo") is the placeholder image, and Image(systemName: "exclamationmark.triangle") is the error image.
Step 4: Adding Options
You can customize the behavior of AmplifyImage by using options. For example, you can set the content mode of the image, or you can enable caching. Follow these steps to add options:
- Create an instance of AmplifyImageOptions:
let options = AmplifyImageOptions(contentMode: .aspectFit, cache: .default)
In the above code, contentMode is set to .aspectFit, which scales the image to fit the view while maintaining its aspect ratio. cache is set to .default, which enables caching of the image.
- Use the AmplifyImage view and pass the options as a parameter:
AmplifyImage(key: "path/to/image.jpg", options: options)
Step 5: Handling Image Loading State
When loading images from a remote server, there may be a delay in retrieving the image. To provide a better user experience, you can display a loading indicator while the image is being retrieved. Follow these steps to handle image loading state:
- Create an instance of AmplifyImageState and set its initial value to .loading:
@State private var imageState: AmplifyImageState = .loading
- Use the AmplifyImage view and pass the state as a parameter:
AmplifyImage(key: "path/to/image.jpg", state: $imageState)
- Use a switch statement to handle the different states of AmplifyImageState:
switch imageState {
case .loading:
ProgressView()
case .success:
AmplifyImage(key: "path/to/image.jpg")
case .failure(let error):
Text(error.localizedDescription)
}
In the above code, ProgressView() is displayed while the image is being retrieved, AmplifyImage(key: "path/to/image.jpg") is displayed when the image is retrieved successfully, and an error message is displayed when an error occurs.
Conclusion
Using AmplifyImage and AWS Amplify Storage, it is easy to display images in your iOS app from a remote server. In this blog post, we walked through the steps to set up AWS Amplify Storage, install AmplifyImage, and display images with AmplifyImage and SwiftUI. We also covered how to add placeholders, error images, and options to customize the behavior of AmplifyImage. Finally, we covered how to handle image loading state to provide a better user experience. With AmplifyImage, displaying images from AWS Amplify Storage in your iOS app has never been easier.