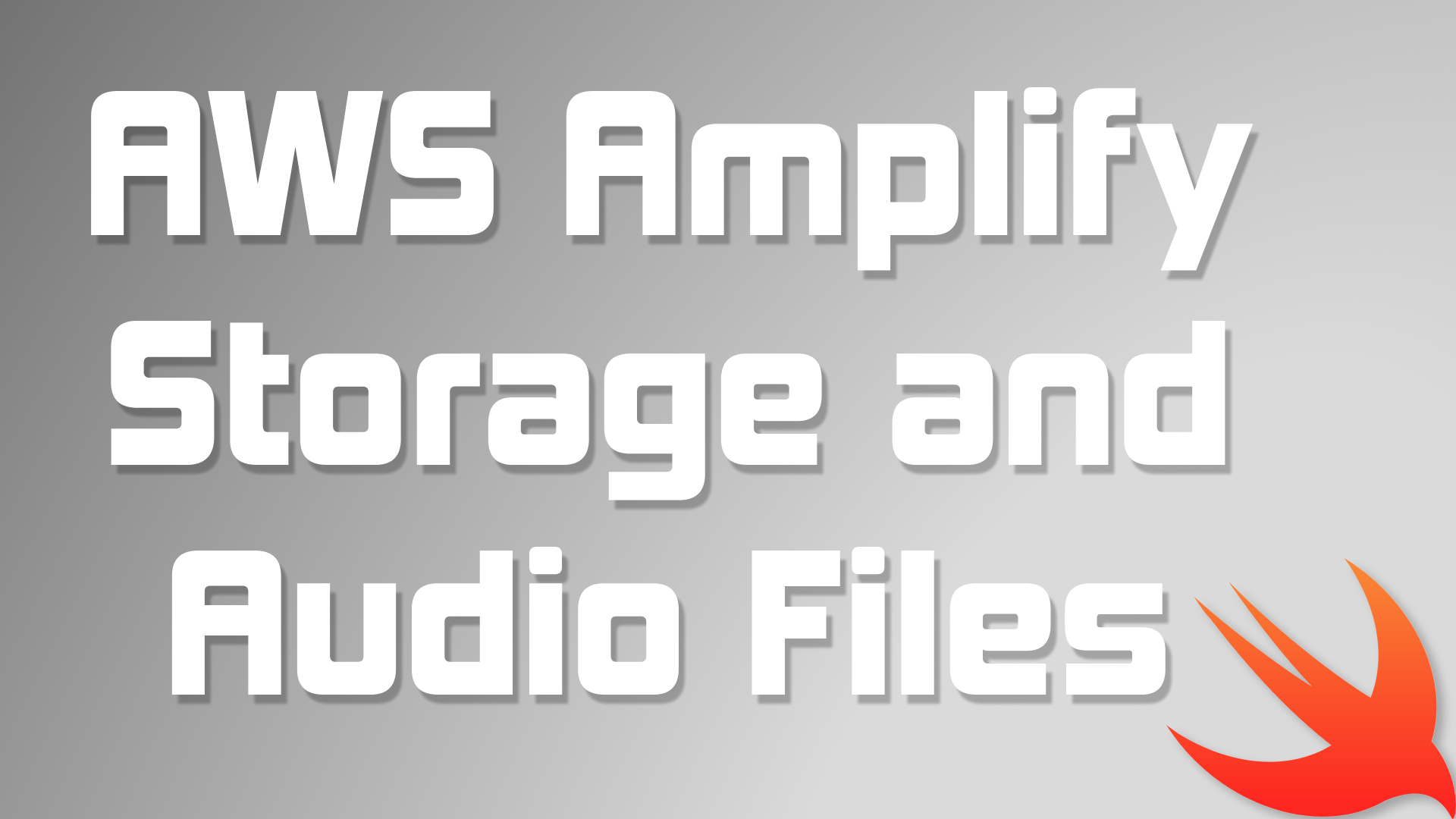
A Comprehensive Guide to Capturing and Storing Audio Files with AWS Amplify Storage on iOS Using SwiftUI
As a mobile app developer, you may find yourself needing to capture and store audio files in your app. Whether it’s for recording memos, voice notes, or audio messages, audio capture is a crucial feature for many iOS apps. In this tutorial, we’ll show you how to capture audio and store the audio file using AWS Amplify Storage on iOS using SwiftUI.
Step 1: Capturing Audio
Now that your environment is set up and your storage resource is configured, you can begin capturing audio. To capture audio, you’ll need to use the AVFoundation framework. Here’s an example of how to set up and start recording audio:
import AVFoundation
class AudioRecorder: NSObject, ObservableObject, AVAudioRecorderDelegate {
var audioRecorder: AVAudioRecorder!
func startRecording() {
let session = AVAudioSession.sharedInstance()
do {
try session.setCategory(.playAndRecord, mode: .default, options: [])
try session.setActive(true)
let url = getDocumentsDirectory().appendingPathComponent("recording.wav")
let settings: [String: Any] = [
AVFormatIDKey: Int(kAudioFormatLinearPCM),
AVSampleRateKey: 44100,
AVNumberOfChannelsKey: 2,
AVEncoderAudioQualityKey: AVAudioQuality.high.rawValue
]
audioRecorder = try AVAudioRecorder(url: url, settings: settings)
audioRecorder.delegate = self
audioRecorder.record()
} catch let error {
print("Error recording audio: \(error.localizedDescription)")
}
}
func stopRecording() {
audioRecorder.stop()
}
func audioRecorderDidFinishRecording(_ recorder: AVAudioRecorder, successfully flag: Bool) {
if !flag {
print("Recording failed")
}
}
func getDocumentsDirectory() -> URL {
let paths = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)
let documentsDirectory = paths[0]
return documentsDirectory
}
}
This code sets up an AudioRecorder class that uses the AVAudioRecorder to start and stop recording audio. It also sets up a function to get the documents directory for the app.
Step 2: Storing Audio with AWS Amplify Storage
Now that you’ve captured the audio, you’ll need to store it using AWS Amplify Storage. Here’s an example of how to upload the audio file to S3 using the Amplify Storage API:
import Amplify
import AWSS3StoragePlugin
class AudioUploader {
func uploadAudio(_ audioURL: URL) {
let key = UUID().uuidString + ".wav"
let uploadTask = Amplify.Storage.uploadFile(
key: key,
local: audioURL
)
Task {
for await progress in await uploadTask.progress {
print("Progress: \(progress)")
}
do {
let data = try await uploadTask.value
print("Upload completed: \(data)")
} catch {
print("Failed to upload file: \(error)")
}
}
}
}
This code sets up an AudioUploader class that uses the Amplify.Storage.uploadFile method to upload the audio file to your configured S3 bucket. The key variable is a unique identifier for the file, which is used to retrieve the file later.
Step 3: Integrating Audio Capture and Storage with SwiftUI
Now that you have captured and stored the audio file, you can integrate it into your SwiftUI app. Here’s an example of how to set up a view that allows the user to record and save an audio file:
import SwiftUI
struct AudioRecorderView: View {
@ObservedObject var audioRecorder = AudioRecorder()
@State private var isRecording = false
var body: some View {
VStack {
Text(isRecording ? "Recording..." : "Tap to Record")
.foregroundColor(.red)
.font(.headline)
.padding()
Button(action: {
if self.audioRecorder.audioRecorder == nil {
self.audioRecorder.startRecording()
} else {
self.audioRecorder.stopRecording()
self.uploadAudio()
}
self.isRecording.toggle()
}) {
Image(systemName: isRecording ? "stop.circle" : "circle.fill")
.resizable()
.aspectRatio(contentMode: .fit)
.frame(width: 100, height: 100)
.foregroundColor(.red)
}
}
}
func uploadAudio() {
guard let audioURL = audioRecorder.audioRecorder.url else { return }
let uploader = AudioUploader()
uploader.uploadAudio(audioURL)
}
}
This code sets up an AudioRecorderView struct that uses the AudioRecorder class to capture and store an audio file. The view displays a button that starts and stops recording, and the uploaded audio file is stored in your configured S3 bucket.
Conclusion
In this tutorial, we have shown you how to capture and store audio files using AWS Amplify Storage on iOS using SwiftUI. We have covered how to configure AWS Amplify Storage, how to capture audio using the AVFoundation framework, and how to upload the audio file to S3 using the Amplify.Storage.uploadFile method. We have also provided a sample SwiftUI view that allows the user to record and save an audio file. With this knowledge, you can now add audio capture and storage functionality to your iOS app with ease.